I recently followed two tutorials from the very endearing Dev Ed on YouTube where he created the very same To Do app in different ways; once in vanilla Javascript and again in React. It only took an hour or two to make each one, but it was a really interesting process.
Here’s what I learned…
1. Vanilla JS is bloody brilliant
But you knew this already, right?
For the vanilla JS version to do everything that the React version does in just one file with ~140 lines of code is incredible when you compare it to the React approach which includes:
- Create React App setup
- JS / JSX split across multiple files
- Dealing with
import
andexport
- 795 dependencies in
node_modules
- A build process
This isn’t me hating on React, but what this process did do for me was highlight how straightforward small, simple projects can be if you don’t let the framework get in your way.
2. …but it’s clear why React was made
While realising how great vanilla JS was for simple projects, re-writing the app with React made it abundantly clear just how much better / easier it can be when it comes to more complex, data-driven, reactive apps.
I think a big part of it comes down to being able to easily build templates of your components in HTML (though I appreciate that it’s actually JSX). Rather than having to build an element line-by-line, one bit at a time, like this:
const completedButton = document.createElement('button')
completedButton.innerHTML = '<i class="fa-solid fa-check"></i>'
completedButton.classList.add('complete-btn')
todoDiv.appendChild(completedButton)
…we can do this instead:
<button onClick={completeHandler} className="complete-btn">
<i className="fa-solid fa-check"></i>
</button>
I appreciate that with vanilla JS we could use template literals to lay out the HTML so that it’s more readable, but it’s still not great (may not get syntax highlighting, etc).
And this really just focuses on one small, basic part of React. Other features such as hooks really take things up a notch.
3. Writing CSS in React doesn’t have to be different
Speaking of React, one thing I’ve not enjoyed in the past is using CSS-in-JS.
Regular CSS is nice and clean, following it’s own object-like syntax of property and value after declaring a selector. With SCSS, you build on that with nesting, functions and the like, but it still very much feels like CSS.
One of my earliest experiences with React introduced the styled-components
library and this very much did not feel like it was the CSS I knew and loved.
// The Button from the last section without the interpolations
const Button = styled.button`
color: palevioletred;
font-size: 1em;
margin: 1em;
padding: 0.25em 1em;
border: 2px solid palevioletred;
border-radius: 3px;
`;
// A new component based on Button, but with some override styles
const TomatoButton = styled(Button)`
color: tomato;
border-color: tomato;
`;
render(
<div>
<Button>Normal Button</Button>
<TomatoButton>Tomato Button</TomatoButton>
</div>
);
I thought styling in React was pretty much only done this way. However, this React to do app project reminded me that we can just write CSS in a separate App.css
file and import
it. Done. Panic over.
Side note: I recognise that my dislike of
styled-components
stemmed from not understanding it properly, and that came from the fact that I was also learning React itself at the time, so it was all very new!
4. Local Storage can be a simple and powerful addition
In both the vanilla JS and the React version, I implemented a feature whereby a user’s to do list is stored their browser’s Local Storage, meaning on reload or revisiting another time the previous to do list would be populated. This is pretty powerful functionality that turns your very simple, static ‘toy’ into feeling far more like a persistent app that might actually be useful. And it’s super simple to implement.
Here’s two functions from our React to do app:
const saveLocalTodos = () => {
localStorage.setItem("todos", JSON.stringify(todos));
};
const getLocalTodos = () => {
if (localStorage.getItem("todos") === null) {
localStorage.setItem("todos", JSON.stringify([]));
} else {
let todoLocal = JSON.parse(localStorage.getItem("todos"));
setTodos(todoLocal);
}
};
As you can see, it mainly boils down to two methods; localStorage.setItem()
to store something and localStorage.getItem()
to retrieve something. Simple!
My point here is that – if looking at the ROI of this feature from a value-added perspective – we’re talking big bucks! Major dollar.
5. Experienced developers make silly mistakes too
There’s a point in the React tutorial video where Ed gets stuck. Just plain stuck.
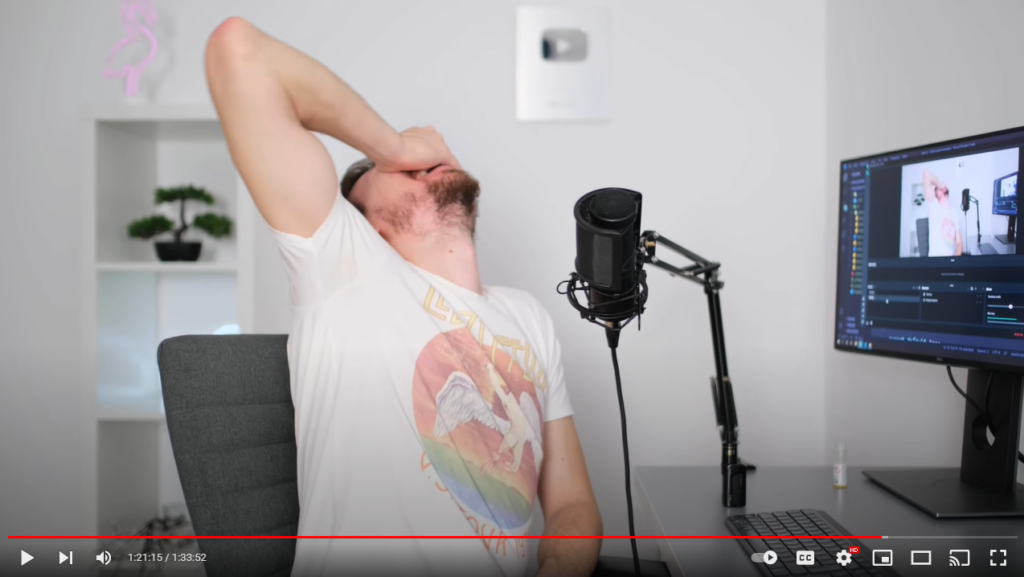
I mean, look at the guy!
And the reason for the error? He passed the props to the wrong component. That’s it. That’s all it was.
Now I’m not saying he’s the most seasoned developer out there, or that he follows the bestest of best practices suitable for a commercial setting, but he’s knowledgeable and experienced enough to do what he’s doing and he got stuck. It was great to see. Obviously not in a sadistic way, but to see that small mistakes like this can trip developers up no matter how long they’ve been coding.
I think I felt even better because I spotted the issue before he did! 😉